2024. 3. 23. 20:29ㆍGUI/tkinter
요약 : 이 튜토리얼에서는 Tkinter 메뉴 표시줄을 만들고, 메뉴 표시줄에 메뉴를 추가하고, 각 메뉴에 메뉴 항목을 추가하는 방법을 배웁니다.
애플리케이션에 많은 기능이 포함되어 있는 경우 더 쉽게 탐색할 수 있도록 메뉴를 사용하여 구성해야 합니다.
일반적으로 메뉴를 사용하여 밀접하게 관련된 작업을 그룹화합니다. 예를 들어 대부분의 텍스트 편집기에서 파일 메뉴를 찾을 수 있습니다.
Tkinter는 기본적으로 메뉴를 지원합니다. 프로그램이 실행하는 대상 플랫폼(예: Windows, macOS 및 Linux)의 모양과 느낌이 포함된 메뉴를 표시합니다.
간단한 메뉴 만들기
먼저 root창을 만들고 제목을 'Menu Demo'로 설정합니다.
root = tk.Tk()
root.title('Menu Demo')
둘째, 메뉴 표시줄을 만들고 root 창의 menu 옵션에 할당합니다.
menubar = Menu(root)
root.config(menu=menubar)
각 최상위 창에는 메뉴 표시줄이 하나만 있을 수 있습니다.
셋째, 컨테이너가 menubar인 파일 메뉴를 만듭니다.
file_menu.add_command(
label='Exit',
command=root.destroy,
)
이 예에서 메뉴 항목의 레이블은 Exit 입니다.
Exit 메뉴 항목을 클릭하면, Python이 자동으로 root.destroy() 메서드를 호출하여 root 창을 닫습니다.
마지막으로 메뉴바에 File 메뉴를 추가합니다.
menubar.add_cascade(
label="File",
menu=file_menu,
underline=0
)
이 underline옵션을 사용하면 키보드 단축키를 만들 수 있습니다. 밑줄을 그어야 하는 문자 위치를 지정합니다.
위치는 0부터 시작됩니다. 이 예에서는 이를 첫 번째 문자인 F로 지정합니다. 그리고 Alt+F 키보드 단축키를 사용하여 F를 선택할 수 있습니다.
모두 합치면 다음과 같습니다.
import tkinter as tk
from tkinter import Menu
# root window
root = tk.Tk()
root.title('Menu Demo')
# create a menubar
menubar = Menu(root)
root.config(menu=menubar)
# create a menu
file_menu = Menu(menubar)
# add a menu item to the menu
file_menu.add_command(
label='Exit',
command=root.destroy
)
# add the File menu to the menubar
menubar.add_cascade(
label="File",
menu=file_menu
)
root.mainloop()
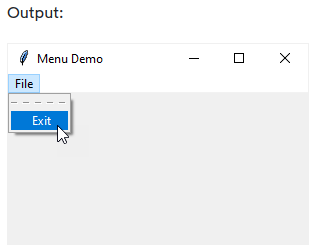
기본적으로 Tkinter는 첫 번째 메뉴 항목 앞에 점선을 추가합니다. 점선을 클릭하면 기본 창에서 다음과 같이 메뉴가 분리됩니다.
점선을 제거하려면, 메뉴의 tearoff 속성을 False로 설정할 수 있습니다.
file_menu = Menu(menubar, tearoff=False)
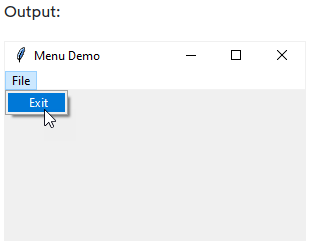
좀 더 복잡한 메뉴 만들기
m다음 프로그램은 메뉴 표시줄을 만들고 메뉴 표시줄에 파일 및 도움말 메뉴를 추가하는 방법을 보여줍니다. 또한 다음 메뉴m에 여러 메뉴 항목을 추가합니다.
from tkinter import Tk, Frame, Menu
# root window
root = Tk()
root.geometry('320x150')
root.title('Menu Demo')
# create a menubar
menubar = Menu(root)
root.config(menu=menubar)
# create the file_menu
file_menu = Menu(
menubar,
tearoff=0
)
# add menu items to the File menu
file_menu.add_command(label='New')
file_menu.add_command(label='Open...')
file_menu.add_command(label='Close')
file_menu.add_separator()
# add Exit menu item
file_menu.add_command(
label='Exit',
command=root.destroy
)
# add the File menu to the menubar
menubar.add_cascade(
label="File",
menu=file_menu
)
# create the Help menu
help_menu = Menu(
menubar,
tearoff=0
)
help_menu.add_command(label='Welcome')
help_menu.add_command(label='About...')
# add the Help menu to the menubar
menubar.add_cascade(
label="Help",
menu=help_menu
)
root.mainloop()
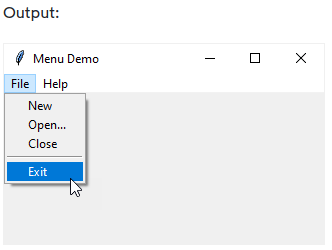
이 프로그램의 유일한 새로운 명령문은 메뉴에 구분 기호를 추가하는 add_separator() 함수를 사용하는 것입니다.
하위 메뉴 추가
다음 프로그램은 메뉴 항목을 Preferences메뉴에 추가 File하고 새 메뉴 항목을 연결하는 하위 메뉴를 만듭니다.
from tkinter import Tk, Menu
# root window
root = Tk()
root.geometry('320x150')
root.title('Menu Demo')
# create a menubar
menubar = Menu(root)
root.config(menu=menubar)
# create the file_menu
file_menu = Menu(
menubar,
tearoff=0
)
# add menu items to the File menu
file_menu.add_command(label='New')
file_menu.add_command(label='Open...')
file_menu.add_command(label='Close')
file_menu.add_separator()
# add a submenu
sub_menu = Menu(file_menu, tearoff=0)
sub_menu.add_command(label='Keyboard Shortcuts')
sub_menu.add_command(label='Color Themes')
# add the File menu to the menubar
file_menu.add_cascade(
label="Preferences",
menu=sub_menu
)
# add Exit menu item
file_menu.add_separator()
file_menu.add_command(
label='Exit',
command=root.destroy
)
menubar.add_cascade(
label="File",
menu=file_menu,
underline=0
)
# create the Help menu
help_menu = Menu(
menubar,
tearoff=0
)
help_menu.add_command(label='Welcome')
help_menu.add_command(label='About...')
# add the Help menu to the menubar
menubar.add_cascade(
label="Help",
menu=help_menu,
underline=0
)
root.mainloop()
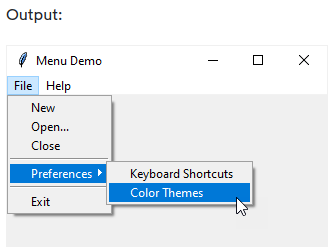
동작 방식.
다음 코드는 File 메뉴에 하위 메뉴를 추가하고, 하위 메뉴를 Preferences 메뉴 항목에 연결합니다.
# add a submenu
sub_menu = Menu(file_menu, tearoff=0)
sub_menu.add_command(label='Keyboard Shortcuts')
sub_menu.add_command(label='Color Themes')
# add the File menu to the menubar
file_menu.add_cascade(
label="Preferences",
menu=sub_menu
)
요약
- 새로운 메뉴를 만들 때 Menu()를 사용하세요.
- 메뉴에 메뉴 항목을 추가하려면 menu.add_command() 메소드를 사용하십시오.
- menubar에 menu을 추가하는 데 menubar.add_cascade(menu_title, menu)를 사용합니다.
- menu에 하위 메뉴를 추가하는 데 menu.add(submenu_title, submenu)를 사용합니다.
'GUI > tkinter' 카테고리의 다른 글
Tkinter OptionMenu (0) | 2024.03.26 |
---|---|
Tkinter Menubutton (0) | 2024.03.24 |
Tkinter Open File Dialog (0) | 2024.03.22 |
Tkinter Color Chooser (0) | 2024.03.21 |
Tkinter askretrycancel (0) | 2024.03.20 |